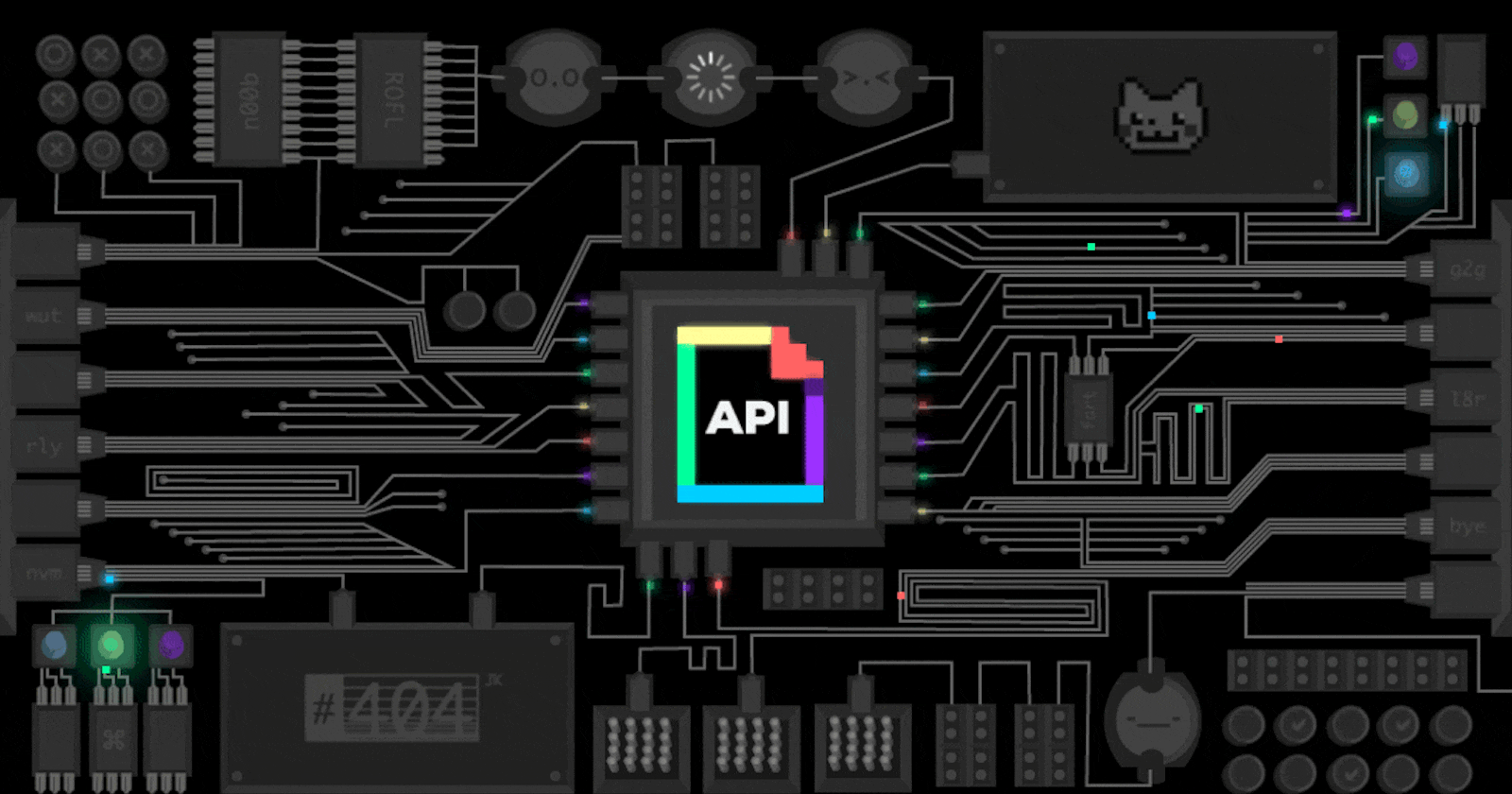
An Introduction to REST APIs: Understanding the Fundamentals and Seeing Them in Action
What is it?
Let us first understand what are APIs - basics
APIs, or application programming interfaces, are an important part of the software industry because they allow different software systems to communicate with each other and exchange data and functionality.
APIs provide a way for different software systems to interoperate, allowing them to work together to create new and powerful applications. For example, a company might expose an API that allows developers to access its product catalog and place orders, which could then be used to build a custom e-commerce platform or a mobile app for purchasing the company's products.
APIs are also used to enable microservices architectures, which are a popular way to build scalable, highly available systems by decomposing a monolithic application into smaller, independent components that can be developed, deployed, and managed separately.
Overall, APIs are an essential tool for enabling software integration and building modern, scalable applications and systems.
Ok, so what does REST mean?
REST, or REpresentational State Transfer, is a software architectural style for designing networked applications. It provides a set of constraints that define how servers and clients can interact with each other to create, read, update, and delete (CRUD) resources.
A REST API is an interface that allows you to access resources through a set of HTTP endpoints, using a fixed set of operations:
POST
: Create a new resourceGET
: Retrieve/Read a resource or a collection of resourcesPUT
: Update an existing resourceDELETE
: Delete a resource
For example, consider an online store that exposes a REST API for retrieving information about products, such as their names, prices, and descriptions. The API might provide the following endpoints:
GET /products
: Retrieve a list of all productsGET /products/{id}
: Retrieve a specific product with the givenid
POST /products
: Create a new productPUT /products/{id}
: Update an existing product with the givenid
DELETE /products/{id}
: Delete a product with the givenid
REST APIs are popular because they are easy to use and understand, and they can be accessed from any platform or programming language that supports HTTP. They are often used to build modern, scalable web applications and microservices.
Need a video to understand better? This might help!
Requests and Responses in a REST API
In a REST API, a client sends an HTTP request to a server to perform a specific action, and the server sends back an HTTP response to indicate the result of the request.
The request message sent by the client typically includes the following elements:
An HTTP method (such as
GET
,POST
,PUT
, orDELETE
) that indicates the desired action to be performedAn HTTP endpoint URL that identifies the specific resource or collection of resources being accessed
An HTTP header that provides additional information about the request, such as the format of the data being sent, the accepted response formats, and authentication credentials
An optional request body that contains data to be sent to the server, such as a JSON payload or a form data
Here is an example of a GET
request in Python using the requests
library:
import requests
response = requests.get("https://api.example.com/products/123") #the URL is imaginary :)
print(response.status_code) # prints 200
print(response.headers) # prints the response headers
print(response.json()) # prints the response body as a JSON object
In this example, the client sends a GET
request to the https://api.example.com/products/123
endpoint to retrieve a product with the ID 123. The requests.get()
function sends the request and returns a Response
object, which contains the server's response. The status_code
attribute of the Response
object indicates the HTTP status code of the response (in this case, 200 OK
), and the headers
attribute contains the response headers. The json()
method of the Response
object parses the response body as a JSON object.
The response message sent by the server typically includes the following elements:
An HTTP status code that indicates the result of the request (such as
200 OK
for a successful request or404 Not Found
if the resource could not be found). Follow this URL to know more about HTTP status codes - https://www.restapitutorial.com/httpstatuscodes.htmlAn HTTP header that provides additional information about the response, such as the format of the data being sent, the server's software version, and caching instructions
An optional response body that contains data returned by the server, such as a JSON payload or HTML content
Here is an example of a simple server that handles GET
requests in Python using the Flask
library:
from flask import Flask, jsonify
app = Flask(__name__)
@app.route("/products/<int:product_id>", methods=["GET"])
def get_product(product_id):
product = get_product_from_database(product_id) # retrieve the product from the database
if product is None:
return "Product not found", 404
return jsonify(product)
In this example, the server defines an endpoint /products/<int:product_id>
that handles GET
requests and expects an int
parameter product_id
. The get_product()
function retrieves the product from the database and returns it as a JSON object using the jsonify()
function. If the product is not found, the function returns an HTTP status code 404 Not Found
.
Overall, requests and responses in a REST API involve sending and receiving HTTP messages with specific methods, URLs, headers, and bodies to perform actions on resources.
Now, here we go - REST API in Action! ๐ฅ
Have you heard of HackerNews? https://news.ycombinator.com/
Hacker News is a community-driven news platform that focuses on topics related to computer science and entrepreneurship. It is operated by Y Combinator, a well-known investment fund and startup incubator. The website is highly respected within the tech industry and is a go-to source for staying up-to-date on the latest trends and happenings in the world of technology and entrepreneurship.
Now, they also have a FREE to use API. Lets play with that!
Here is the URL to the HackerNews API documentation - https://hackernews.api-docs.io/v0/overview/introduction
import requests
# Make a GET request to the API to retrieve the top 500 stories
response = requests.get("https://hacker-news.firebaseio.com/v0/topstories.json")
# Check the status code of the response
if response.status_code == 200:
# Print the titles of the top 10 stories
story_ids = response.json()[:10]
for story_id in story_ids:
story_response = requests.get(f"https://hacker-news.firebaseio.com/v0/item/{story_id}.json")
story = story_response.json()
print(story["title"])
else:
# Print the error message
print(f"Error: {response.status_code} {response.reason}")
In this example, the client sends a GET
request to the https://hacker-news.firebaseio.com/v0/topstories.json
endpoint to retrieve the top 500 stories on Hacker News. The requests.get()
function sends the request and returns a Response
object, which contains the server's response. If the request was successful (status code 200 OK
), the json()
method of the Response
object parses the response body as a JSON array of story IDs and retrieves the first 10 stories using separate GET
requests to the /item/{id}.json
endpoint. The client prints the titles of the stories. If the request was not successful, the client prints an error message with the status code and the reason.
TRY it out here!
This is just a simple example of how you can use the Hacker News API to retrieve data from the server. You can use similar techniques to perform other actions, such as posting comments or voting on stories, by using different HTTP methods and sending data in the request body.
Keep in mind that this is just one way to use the Hacker News API, and the specific details of how to interact with the API will depend on the API's documentation and the requirements of the server.
That's all for now, folks. Enjoy!